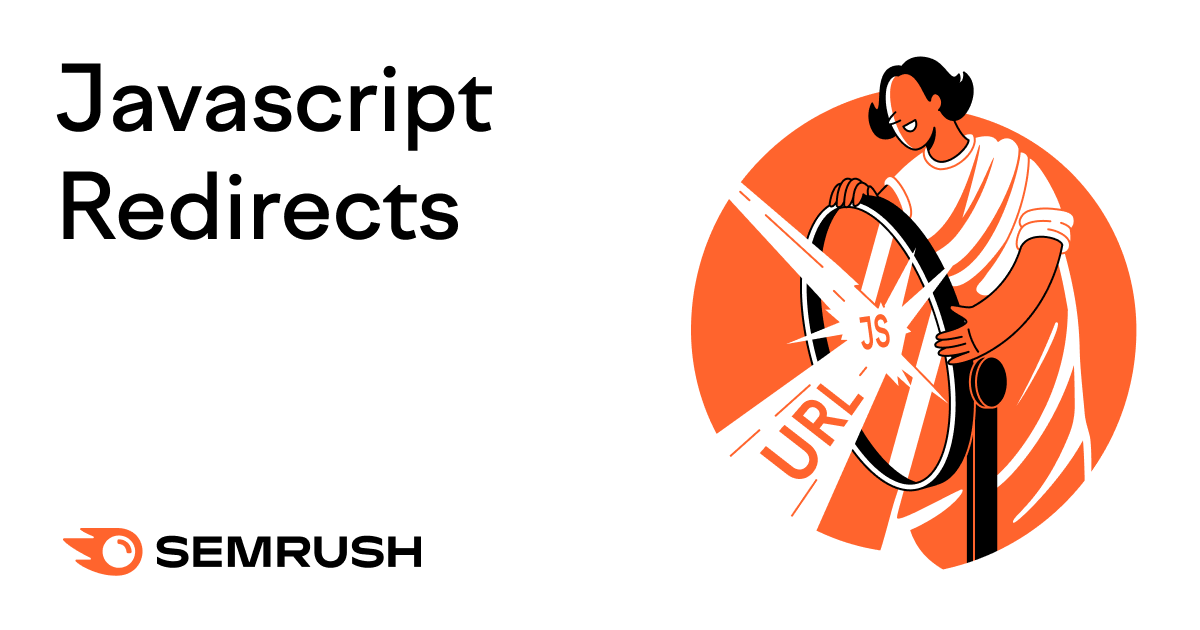
How to Redirect to a New URL
- Digital MarketingNews
- August 7, 2023
- No Comment
- 113
[ad_1]
A JavaScript redirect makes use of the programming language JavaScript (JS) to ship customers from one URL to a different.
You should use a JS redirect to redirect customers to a affirmation web page after they submit a type or to supply fallback redirection if a web page will get deleted, for instance.
However they aren’t typically not advisable for website positioning as a result of engines like google can have problem crawling and indexing websites that depend on them.
On this article, we’ll talk about making a redirect with JS and counsel options.
Tips on how to Redirect with JavaScript
There are three fundamental methods to redirect to a different URL with JavaScript:
- window.location.href
- location.assign()
- location.change()
Set a New window.location.href Property
You should use the window.location.href property in JavaScript to get or set the URL of the present webpage.
The usage of “window.location.href” for redirection simulates the motion of clicking on a hyperlink. It provides a brand new entry to the browser’s session historical past. This enables customers to make use of the “again” button to return to the earlier web page.
To redirect a consumer to a distinct URL, you’ll be able to assign a brand new URL to this property.
The syntax is:
window.location.href = ‘https://exampleURL.com/’;
When tied to a consumer interaction-triggered change, equivalent to a button click on, this could redirect the consumer to “https://exampleURL.com/”
How?
You must add the code within <script> tags within the <head> of your webpage and add an “occasion handler” to a button.
Here is how you would try this:
<html>
<head>
<script>
operate myFunction()
window.location.href = "https://exampleURL.com/";
</script>
</head>
<physique>
<h2>Redirect to a Webpage</h2>
<p>The location.href methodology redirects the consumer to a new webpage:</p>
<button onclick="myFunction()">Redirect</button>
</physique>
</html>
When the consumer clicks the “Redirect” button, it triggers the onclick occasion handler, which calls the myFunction() operate. The execution of myFunction() modifications the worth of “location.href” to “https://exampleURL.com/”, which instructs the browser to navigate to that URL.
Let’s run by means of two different use instances for JavaScript redirects.
The instance under demonstrates utilizing setTimeout() for a JavaScript redirect.
It is a built-in JavaScript operate that allows you to run one other operate after a sure period of time (10 seconds within the instance under).
<html>
<head>
<script sort="textual content/JavaScript">
operate Redirect()
window.location = "https://exampleURL.com/";
doc.write("You will be redirected to the fundamental web page in 10 seconds.");
setTimeout(operate()
Redirect();
, 10000);
</script>
</head>
</html>
One other use case might embody redirecting a consumer based mostly on the browser they’re utilizing.
<html>
<head>
<title>Your Web page Title</title>
<script sort="textual content/javascript">
window.onload = operate()
var userAgent = navigator.userAgent.toLowerCase();
if (userAgent.consists of('chrome'))
window.location.href = "http://www.location.com/chrome";
else if (userAgent.consists of('safari'))
window.location.href = "http://www.location.com/safari";
else
window.location.href = "http://www.location.com/different";
</script>
</head>
<physique>
<!-- Your physique content material goes right here -->
</physique>
</html>
High tip: Keep away from creating JavaScript redirects within the header with out tying them to consumer actions to forestall potential redirect loops (extra on this later). To forestall customers from utilizing the “again” button, use “window.location.change.”
Redirect Utilizing the situation.assign() Technique
You should use “window.location.assign” as a substitute for “window.location.href” for redirects.
The syntax is:
window.location.assign(‘https://www.exampleURL.com/’);
Right here’s what it appears like within a script tag:
<script sort="textual content/JavaScript">
operate Redirect()
window.location.assign("https://exampleURL.com/");
</script>
From a consumer’s perspective, a redirect utilizing “window.location.assign()” appears the identical as utilizing “window.location.href”.
It’s because:
- They each create a brand new entry within the shopping session historical past (much like clicking on a hyperlink)
- They each allow customers to return and look at the earlier web page
Equally to “window.location.href,” it directs the browser to load the desired URL and add this new web page to the session historical past—permitting customers to make use of the browser’s “again” button to return to the earlier web page.
Nevertheless, “window.location.assign()” calls a operate to show the web page situated on the specified URL. Relying in your browser, this may be slower than merely setting a brand new href property utilizing “window.location.href”.
However on the whole, each “window.location.assign()” and altering the situation.href property ought to work simply fantastic. The selection between them is usually a matter of non-public desire and coding model.
Redirect Utilizing the situation.change() Technique
Like “window.location.assign()”, “window.location.change()” calls a operate to show a brand new doc on the specified URL.
The syntax is:
window.location.change('https://www.exampleURL.com/');
Right here’s what it appears like within a script tag:
<script sort="textual content/JavaScript">
operate Redirect()
window.location.change("https://exampleURL.com/");
</script>
Not like setting a brand new location property or utilizing “window.location.assign()” the change methodology doesn’t create a brand new entry in your session historical past.
As an alternative, it replaces the present entry. Which means customers can’t click on the “again” button and return to the earlier, pre-redirect web page.
Why would you wish to do that?
Examples of the place you could use the “window.location.change()” methodology embody:
- Login redirects: After profitable login, customers are directed to the primary web site content material, and the login web page is deliberately omitted from the browser historical past to forestall unintentional return by means of the “again” button
- Person authentication: To redirect unauthenticated customers to a login web page, blocking “again” button entry to the earlier web page
- Kind submission success: Publish-form submission, “window.location.change()” can navigate customers to a hit web page, stopping type resubmission
- Geolocation: Based mostly on the geolocation of a consumer, you’ll be able to redirect them to a model of your web site that is extra appropriate for his or her location, like a web site that is translated to their language
To recap:
Use “window.location.change()” if you don’t need the consumer to have the ability to return to the unique web page utilizing the “again” button.
Use “window.location.assign()” or “window.location.href” if you need the consumer to have the ability to return to the unique web page utilizing the “again” button.
However whilst you can redirect customers to a brand new web page utilizing JavaScript strategies like “window.location.assign()” or “window.location.href”, server-side redirects, equivalent to HTTP redirects, are sometimes advisable as a substitute.
Observe: When you’re simply beginning out or wish to observe JavaScript, you’ll be able to go to an internet site like W3Schools, which affords an interactive W3Schools Tryit Editor for JavaScript, HTML, CSS, and extra:
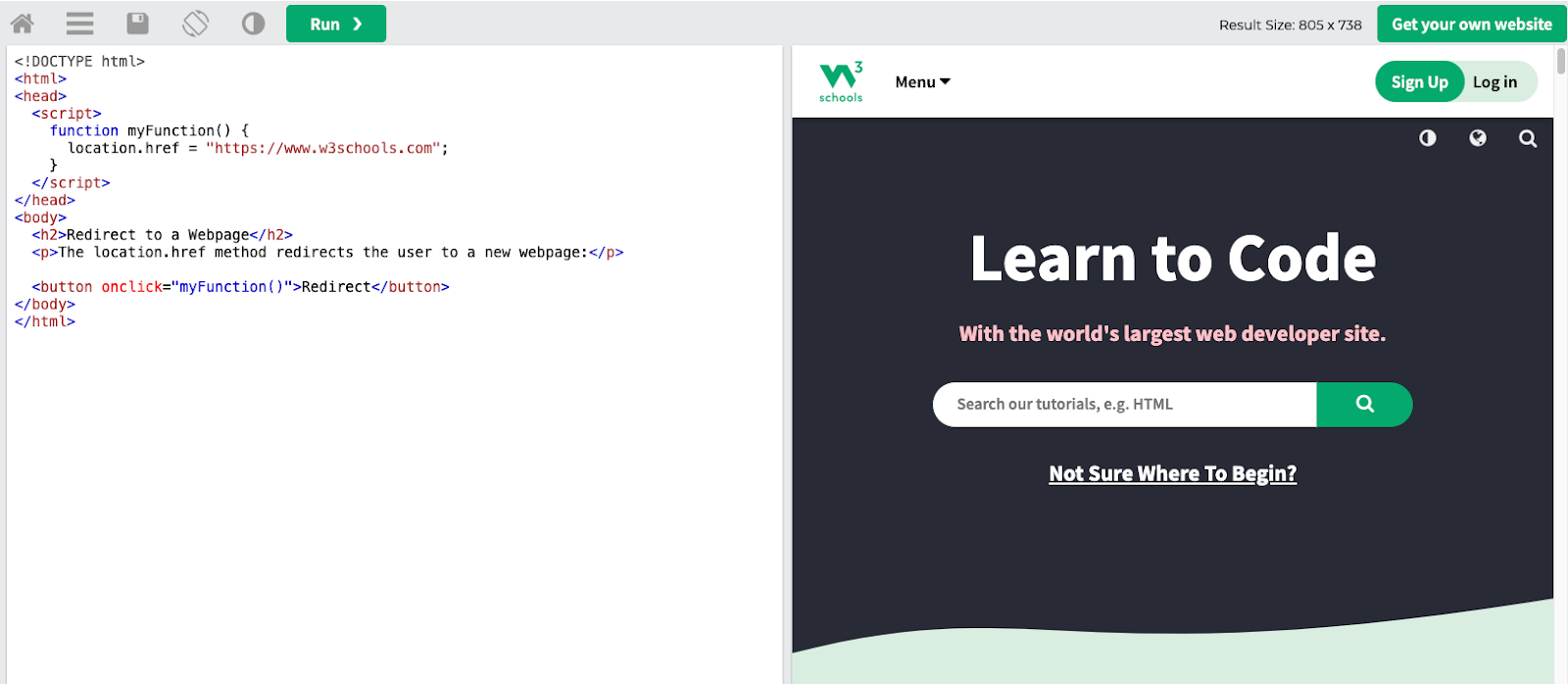
How Google Understands and Processes JavaScript Redirects
Google processes JavaScript redirects the identical manner it executes and renders JavaScript when crawling and indexing web sites.
Right here’s what that appears like, according to Google:
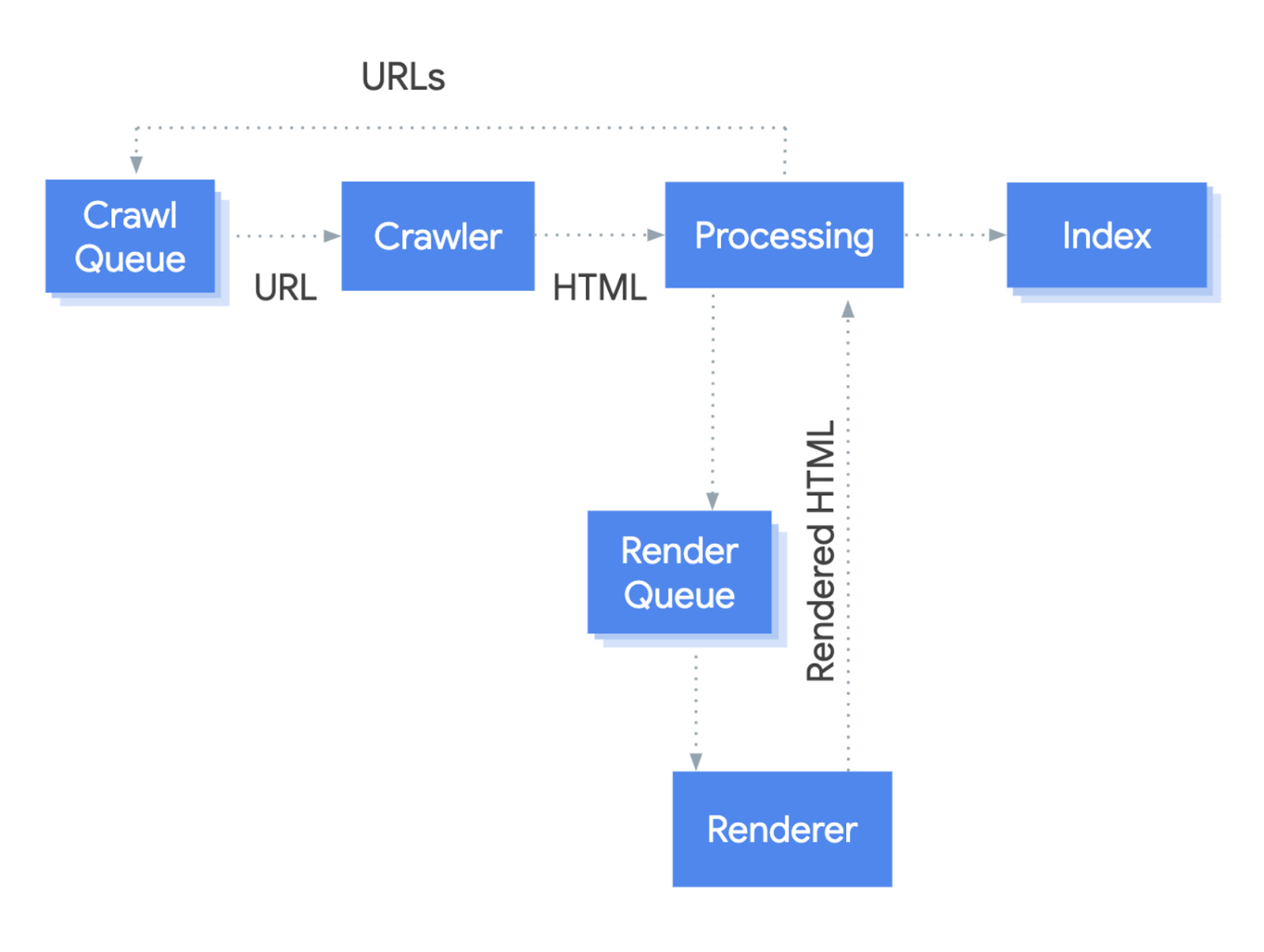
Google’s net crawler, Googlebot, initially indexes a webpage’s HTML content material. It locations any detected JavaScript, together with redirects, right into a “Render Queue” for later processing.
This two-step course of helps Google rapidly add essential content material to its search index. JavaScript is handled later as a result of Google wants extra sources to course of it.
Be taught extra: JavaScript SEO: How to Optimize JS for Search Engines
However, regardless of being able to dealing with JavaScript redirects, Google advises you to avoid using them if attainable as a consequence of their excessive useful resource consumption and processing time.
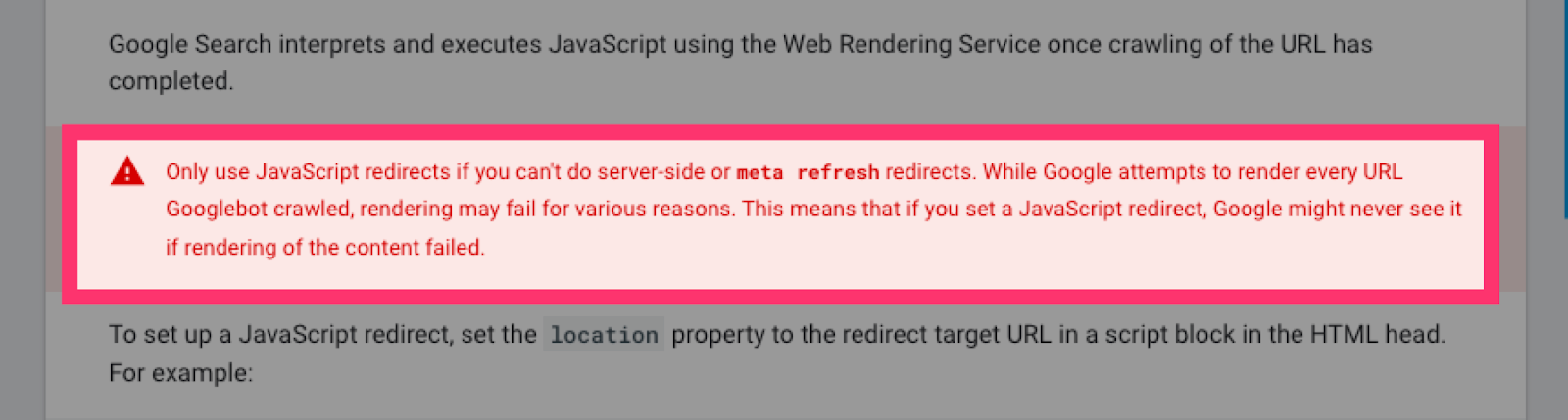
This was additionally echoed by John Mueller in a June 2022 SEO Office Hours video (11:25).

So JavaScript-triggered consumer actions, like a redirect after a button or hyperlink click on, might go unnoticed. Therefore Google’s preference for server-side redirects, equivalent to HTTP redirects—which supply better effectivity, reliability, and search engine optimization (website positioning) advantages.
HTTP redirects have a number of benefits over JavaScript redirects, together with:
- Constant navigation historical past: HTTP redirects do not intrude with the consumer’s navigation historical past. Not like the “location.change()” methodology, customers can nonetheless use the again button to return to earlier pages.
- website positioning friendliness: HTTP redirects present express directions for engines like google when pages transfer, which helps preserve a web site’s SEO ranking. For instance, not like a JS redirect, a 301 redirect tells engines like google that content material has completely moved to a brand new URL.
- Efficiency: HTTP redirects happen on the server stage earlier than the content material is distributed to the browser, which may make them sooner than JavaScript redirects and enhance your page speed
- Reliability: Customers might select to disable JavaScript for safety and privateness causes. HTTP redirects will work even when a consumer has JavaScript disabled, making them extra dependable.
Be taught extra: The Ultimate Guide to Redirects: URL Redirections Explained
Tips on how to Determine JavaScript and HTTP Redirect Points
When working with JavaScript and HTTP redirects, the commonest points you may encounter embody:
- Redirect chains and loops
- Linking to pages with redirects
Redirect Chains and Loops
You may create a redirect chain or loop with out realizing it when coping with redirects.
What’s a redirect chain?
A redirect chain is when it’s good to “hop” by means of a number of URLs earlier than reaching the ultimate one.
For instance, think about that you just initially have a web page with URL A. You then resolve to redirect URL A to a brand new URL B. At some later level, you select to redirect URL B to URL C.
On this case, you’ve got created a redirect chain from URL A to URL B to URL C.
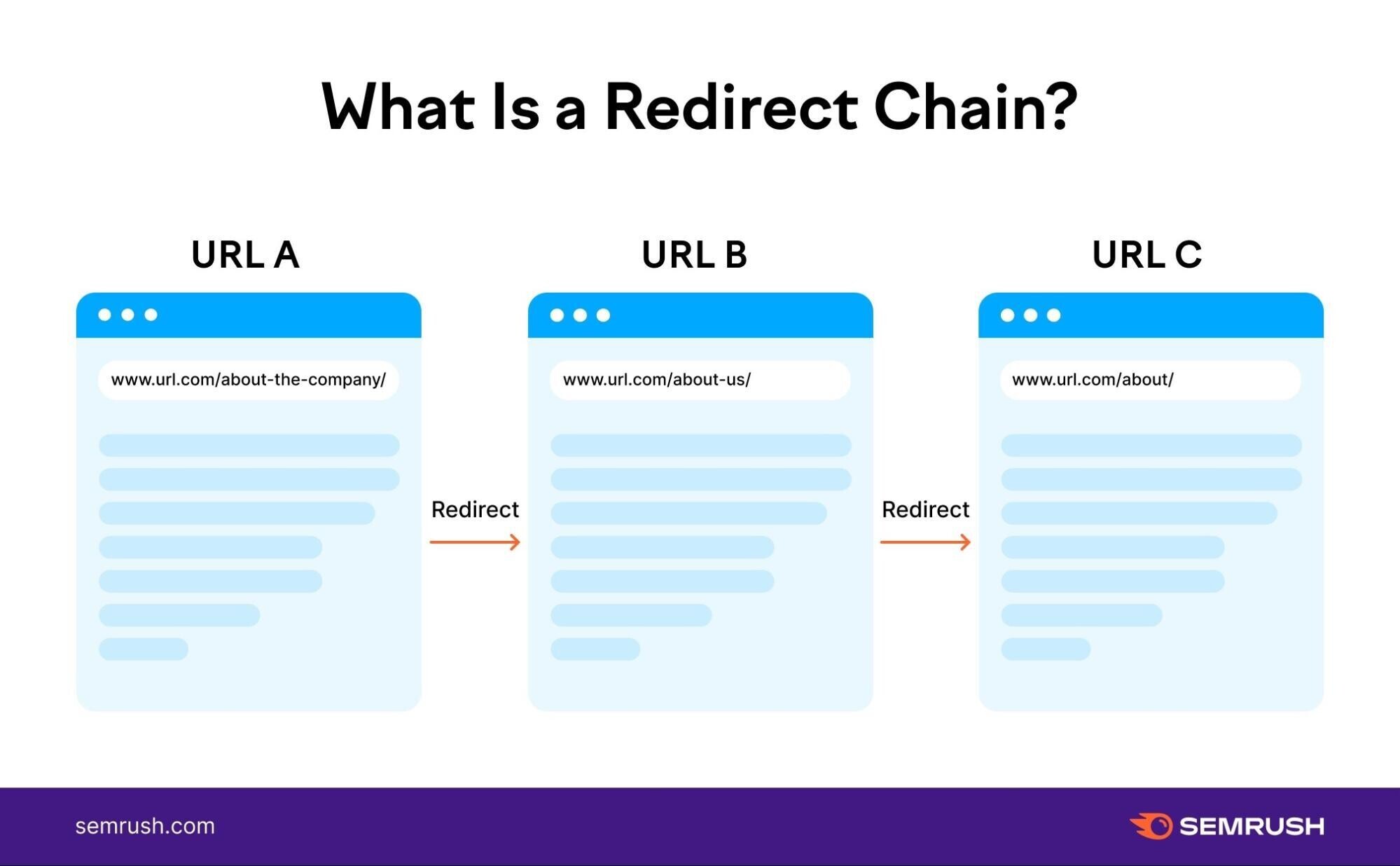
Google can comfortably deal with as much as 10 redirect “hops.”
However too many redirect chains could cause points with website crawling, probably rising the time it takes a page to load. This might additionally negatively influence your website positioning rating and annoy your customers.
To resolve this drawback, redirect URL A to URL C (bypassing URL B).
Right here’s what the redirect chain ought to seem like:
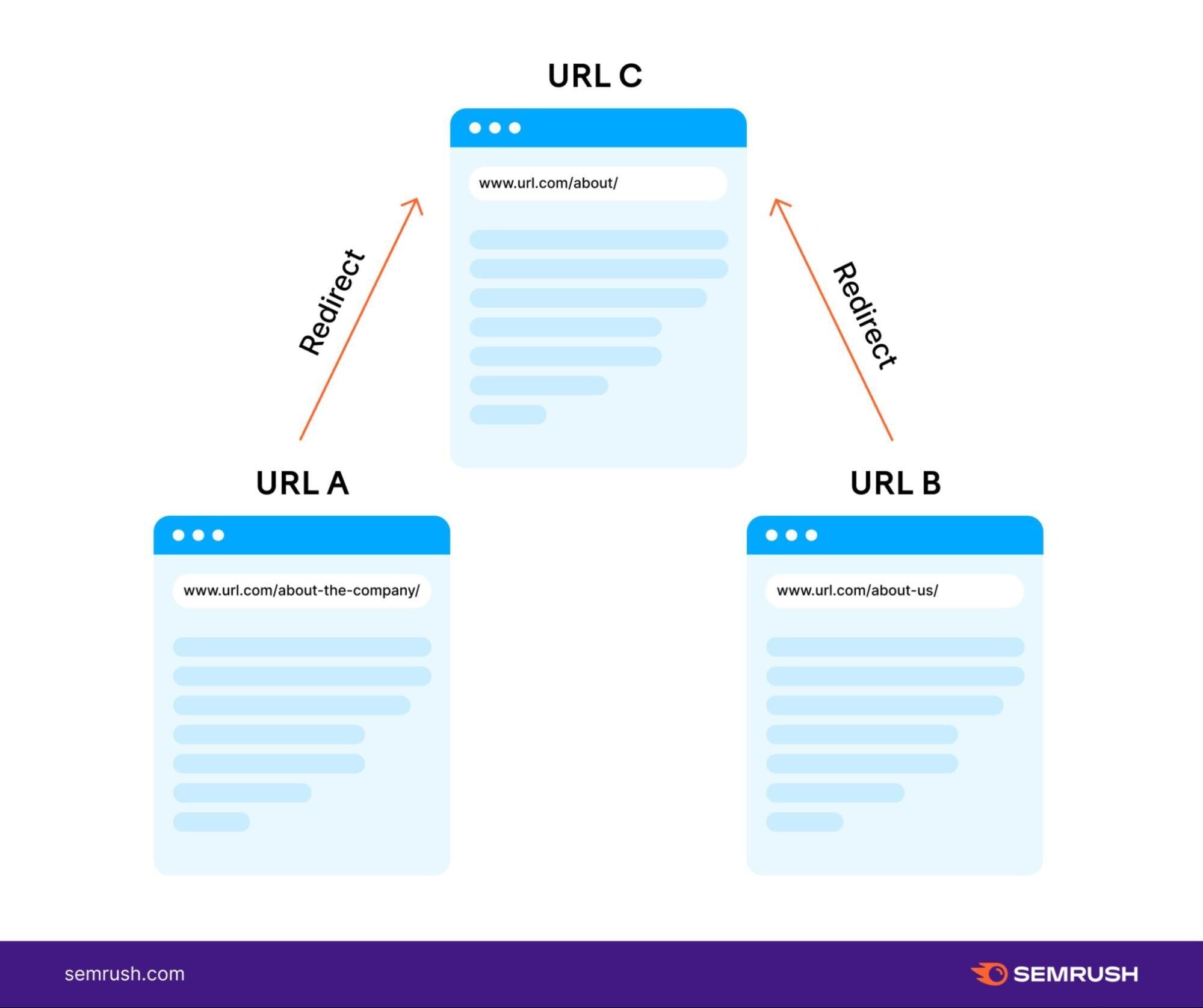
What’s a redirect loop?
A redirect loop occurs when a URL redirects to a distinct URL, after which that second URL redirects again to the primary one, making a unending cycle of redirects.
For instance:
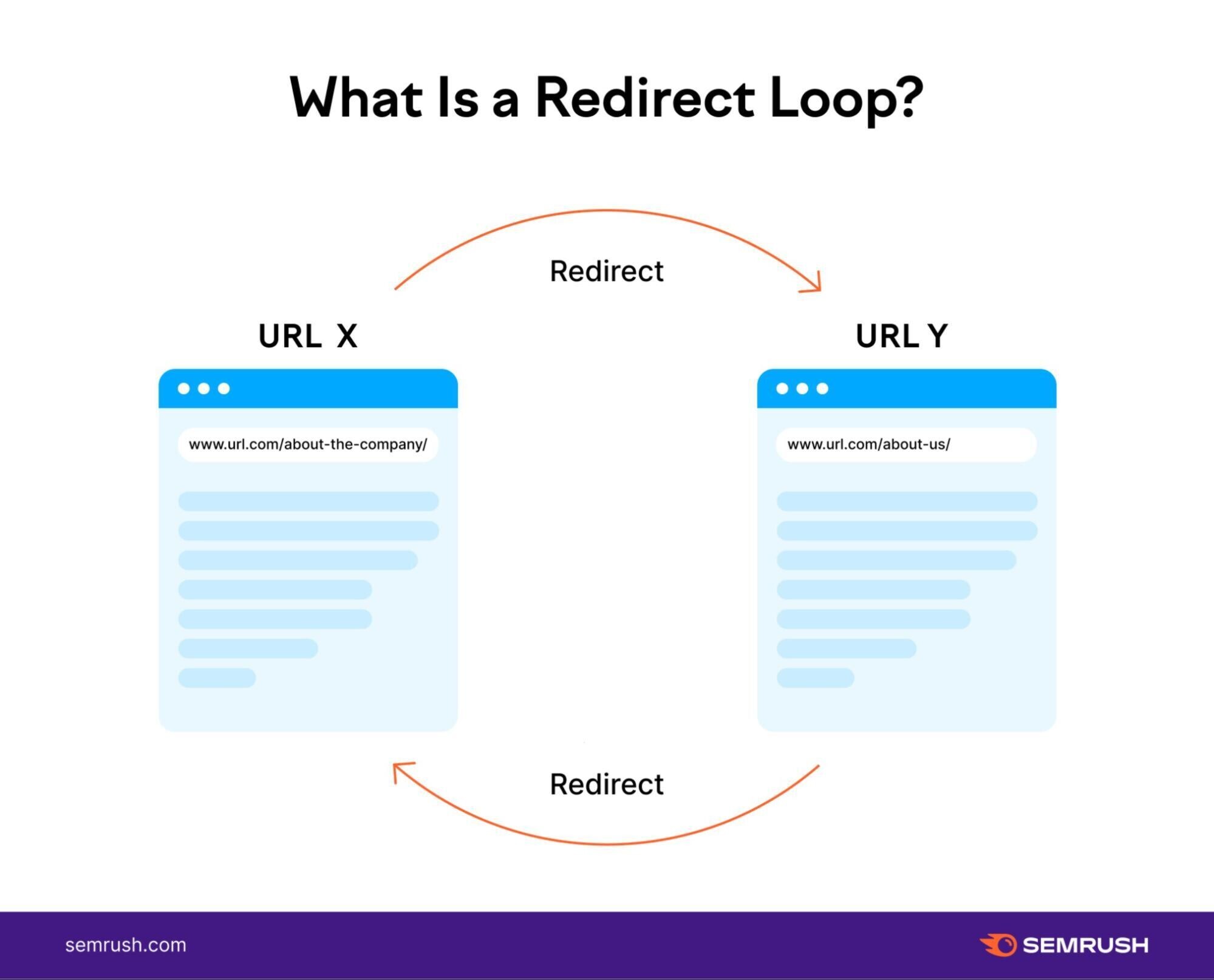
Any such redirection is damaged and fails to correctly direct guests or engines like google to the supposed URL.
To repair redirect loops, determine the “appropriate” web page and make sure the different web page redirects to it. Then, eliminate any additional redirects which are inflicting the loop.
Semrush’s Site Audit tool might help you detect each HTTP and JavaScript redirect chains.
How?
First, create a project or click on on an present one you wish to take a look at.
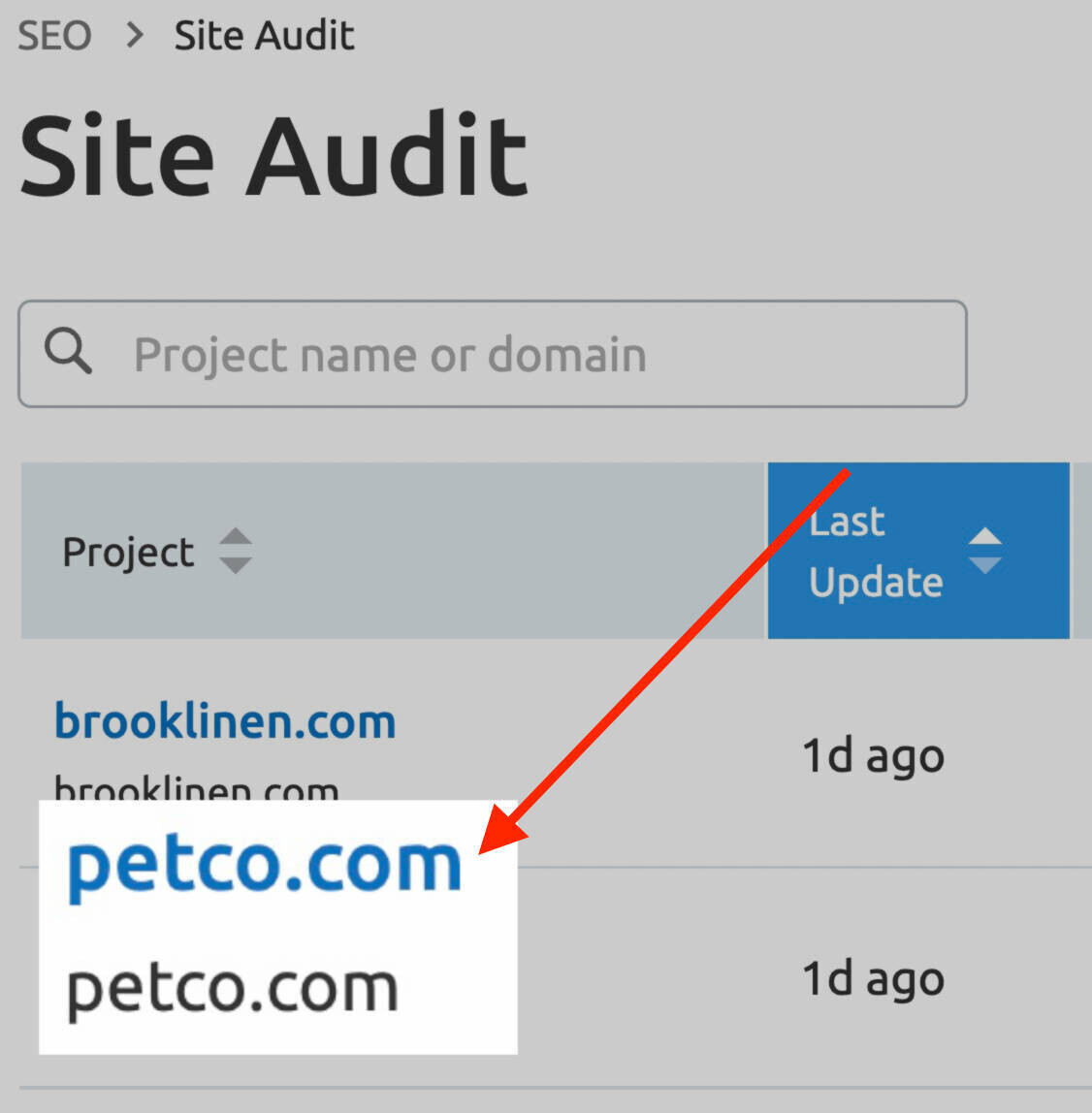
Choose the “Points” tab and seek for “redirect chain” by coming into it into the search bar.
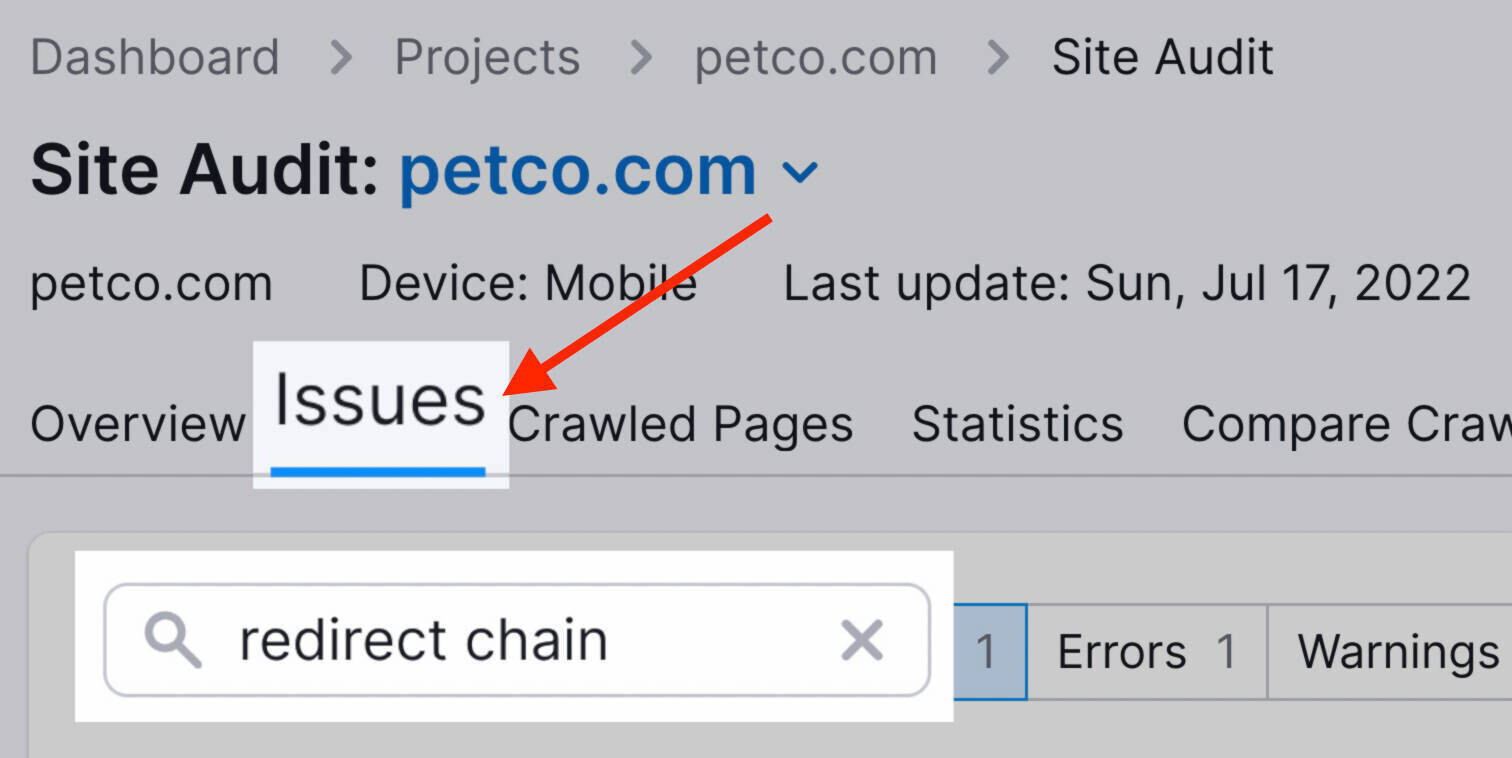
Click on on “# redirect chains and loops.”
This will provide you with a report with all of your web site’s redirect chain or loop errors.
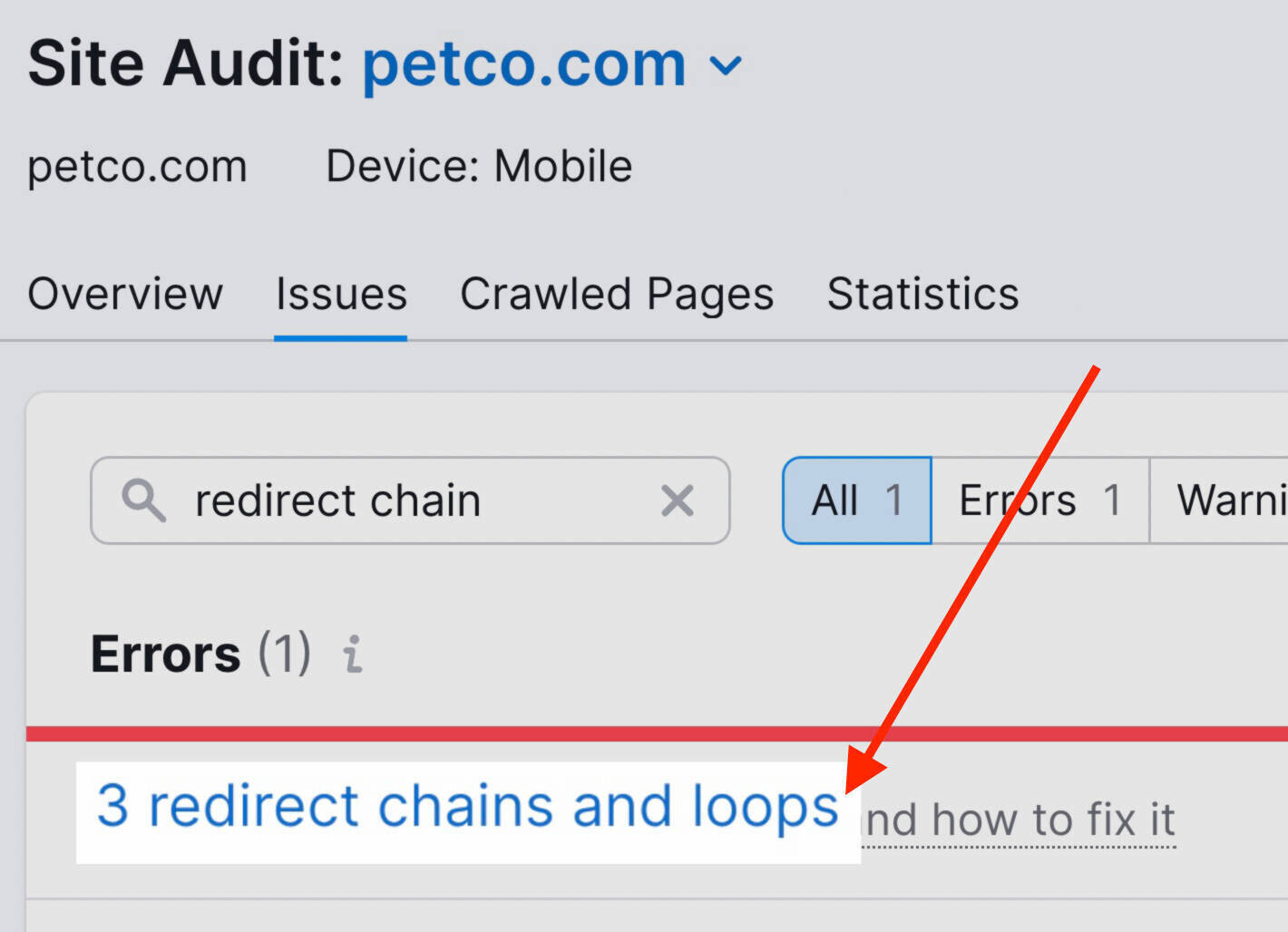
The report consists of particulars such because the web page URL with the redirect hyperlink, the kind of redirect, and the “size” of the chain or loop.
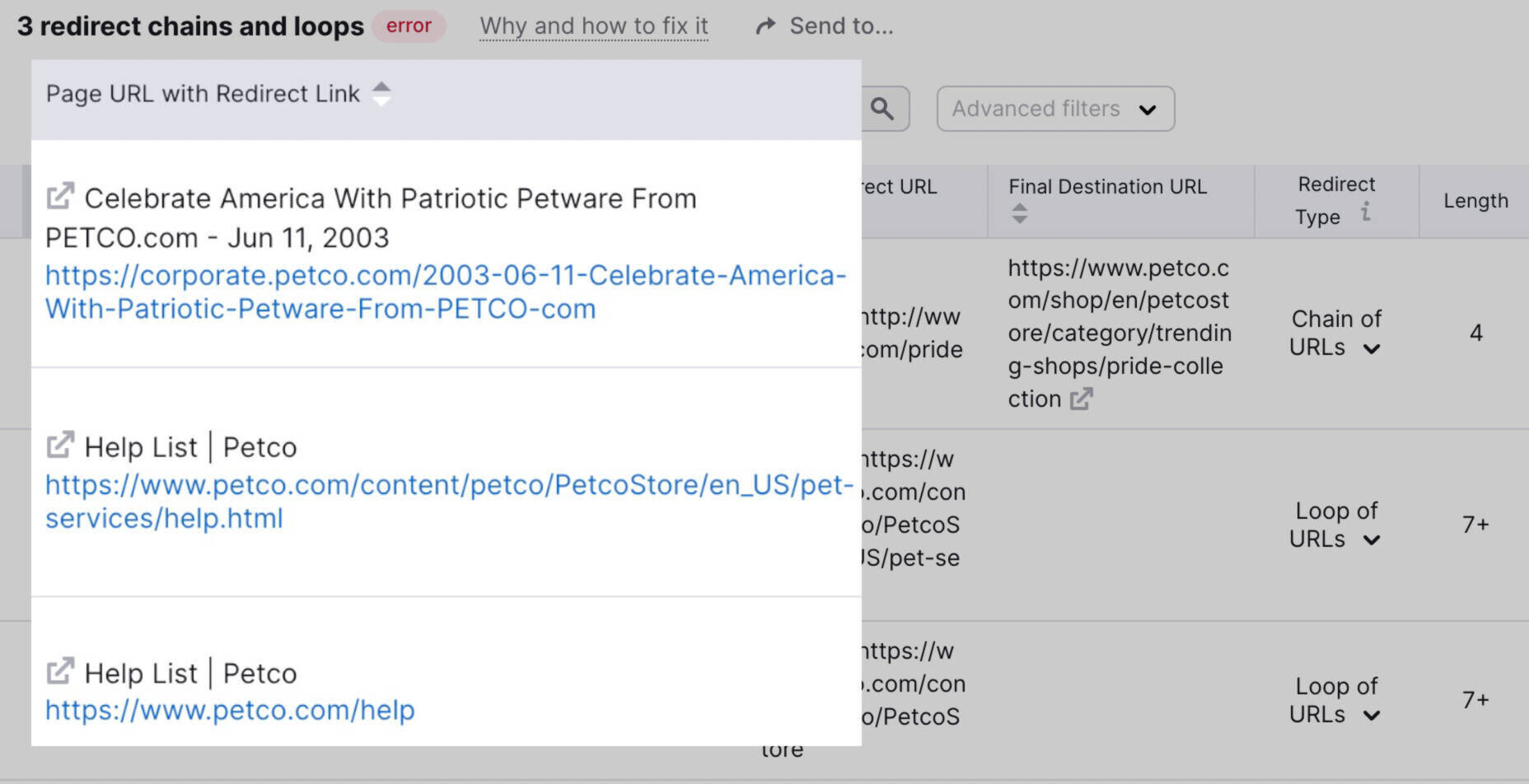
You possibly can then signal into your content material administration system (CMS) and repair every subject.
Linking to Pages with Redirects
Suppose you redirect an outdated web page to a brand new one. Some pages in your web site may nonetheless hyperlink to the outdated web page.
If that is the case, customers shall be directed to your outdated hyperlink, and from there, they are going to be redirected to the brand new URL.
Customers won’t even discover this taking place. Nevertheless, this extra redirect can add as much as a “redirect chain” if you happen to fail to handle the difficulty the primary time.
Here is how this drawback seems:
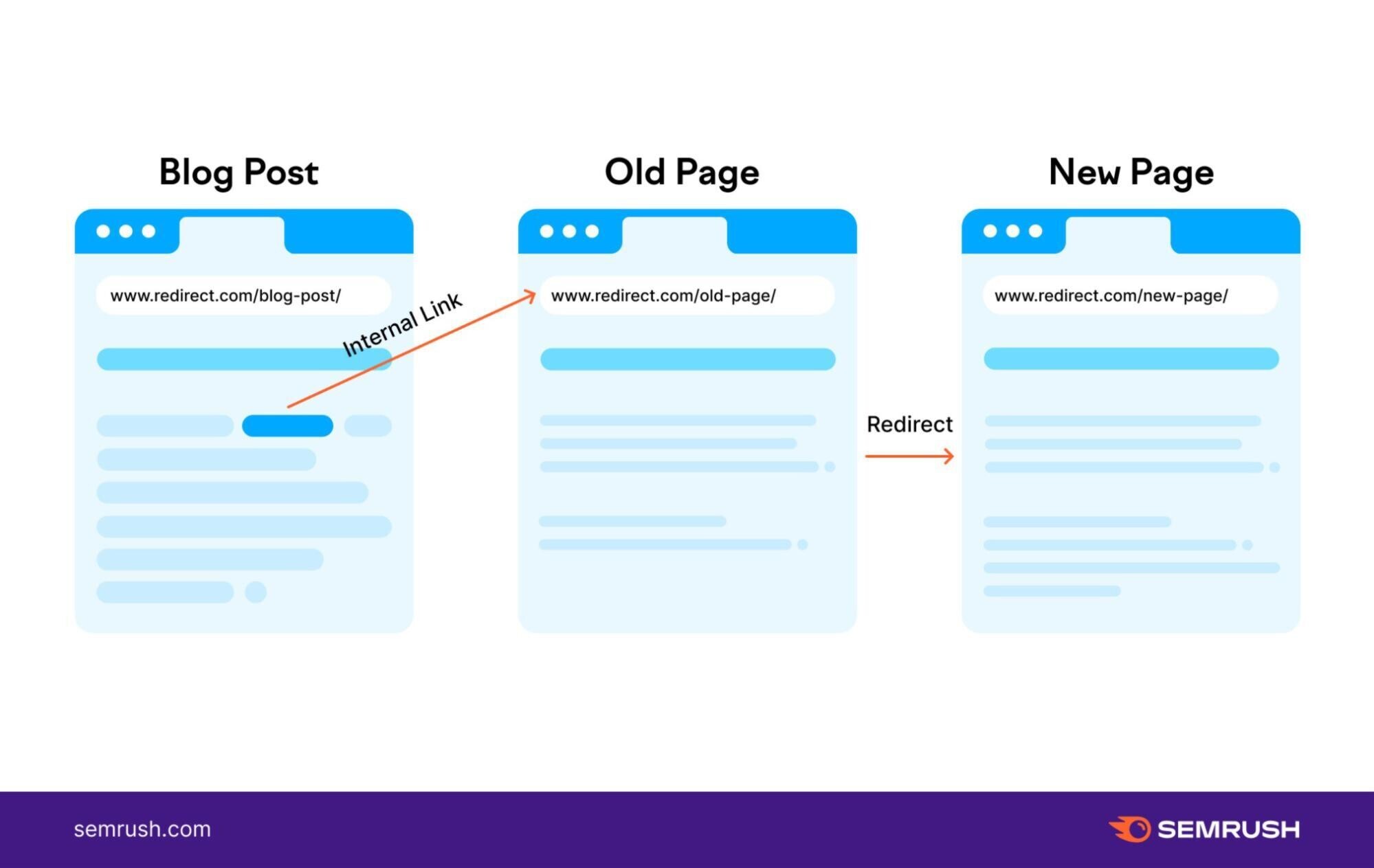
Updating the outdated inside hyperlinks to hyperlink on to your new web page’s URL is finest.
That is the way it ought to look:
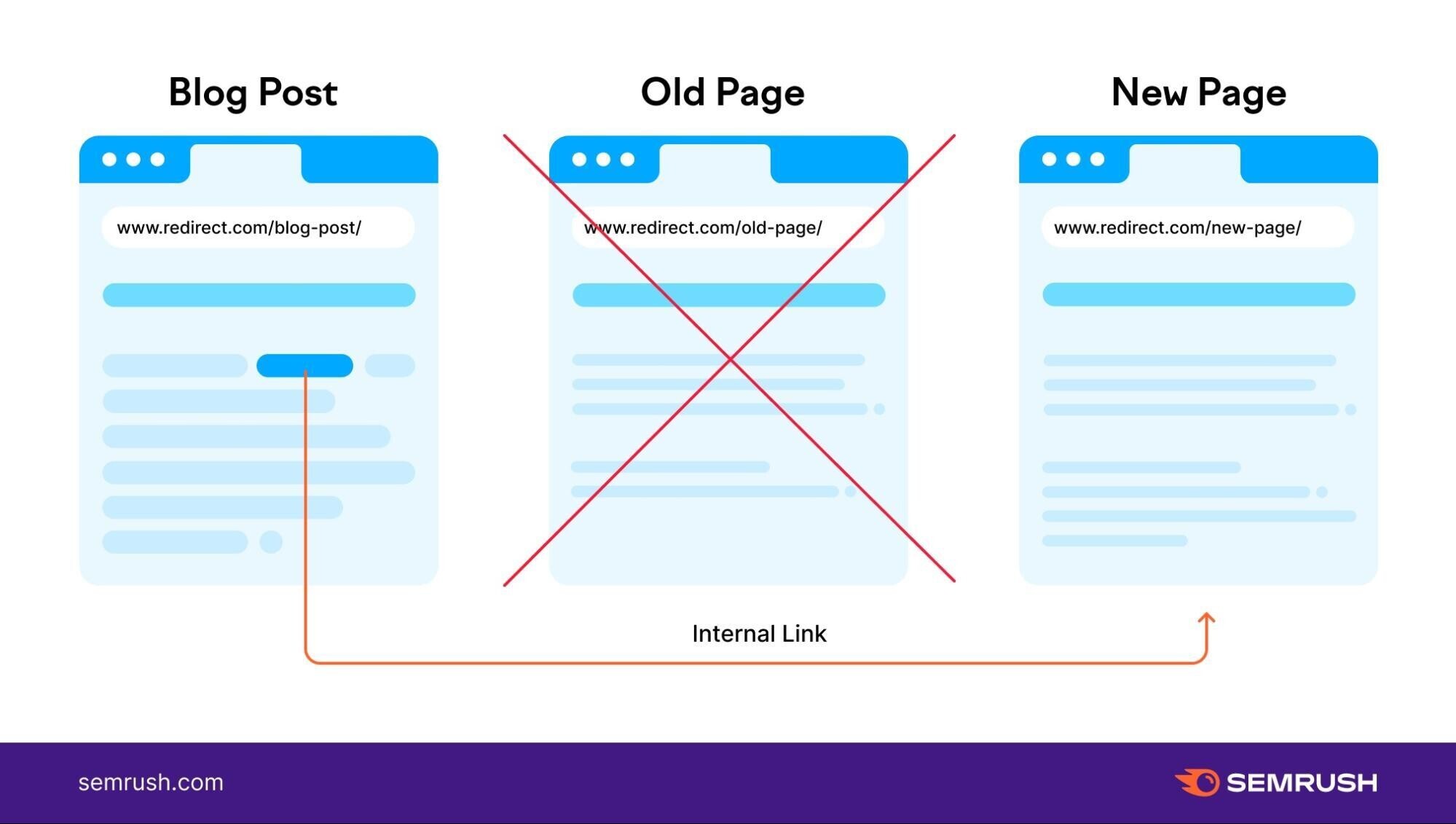
However how will you discover hyperlinks pointing to redirects?
You are able to do this by going to the “Crawled Pages” tab of the Website Audit software:
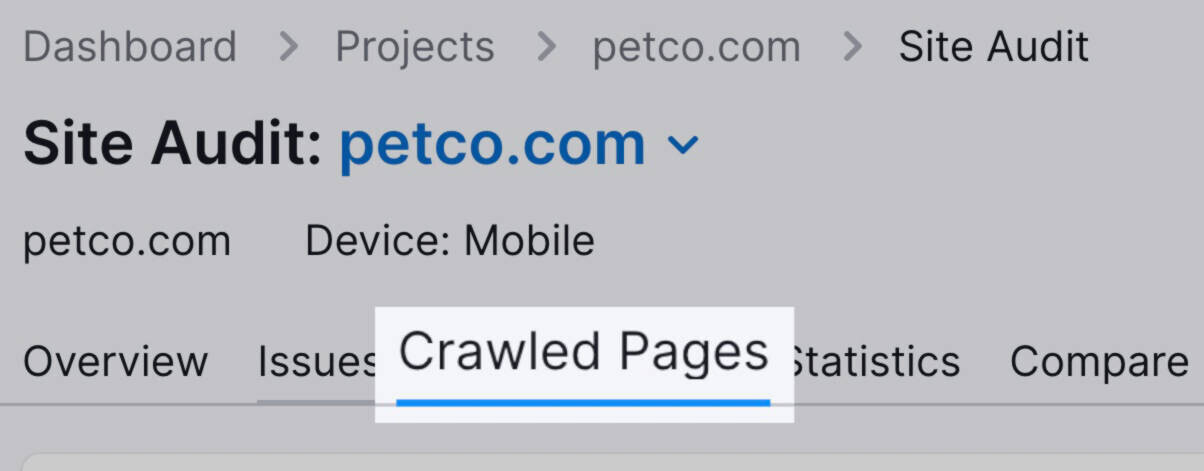
Subsequent, enter your outdated URL (the one which’s being redirected) into the “Filter by Web page URL” subject.
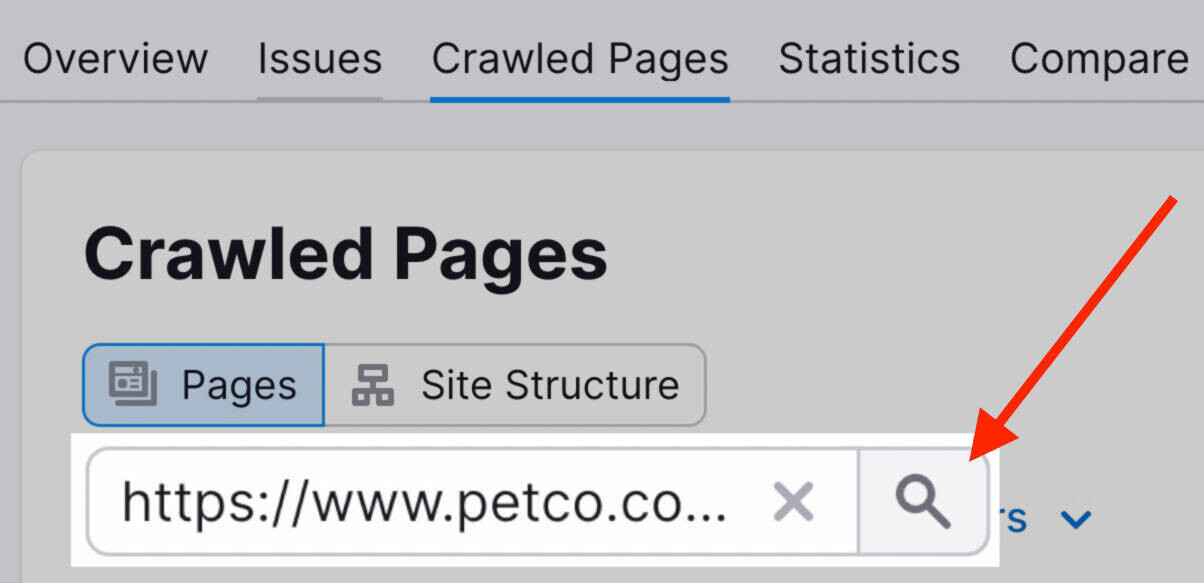
Press “Enter” to see a report for the URL you simply entered.
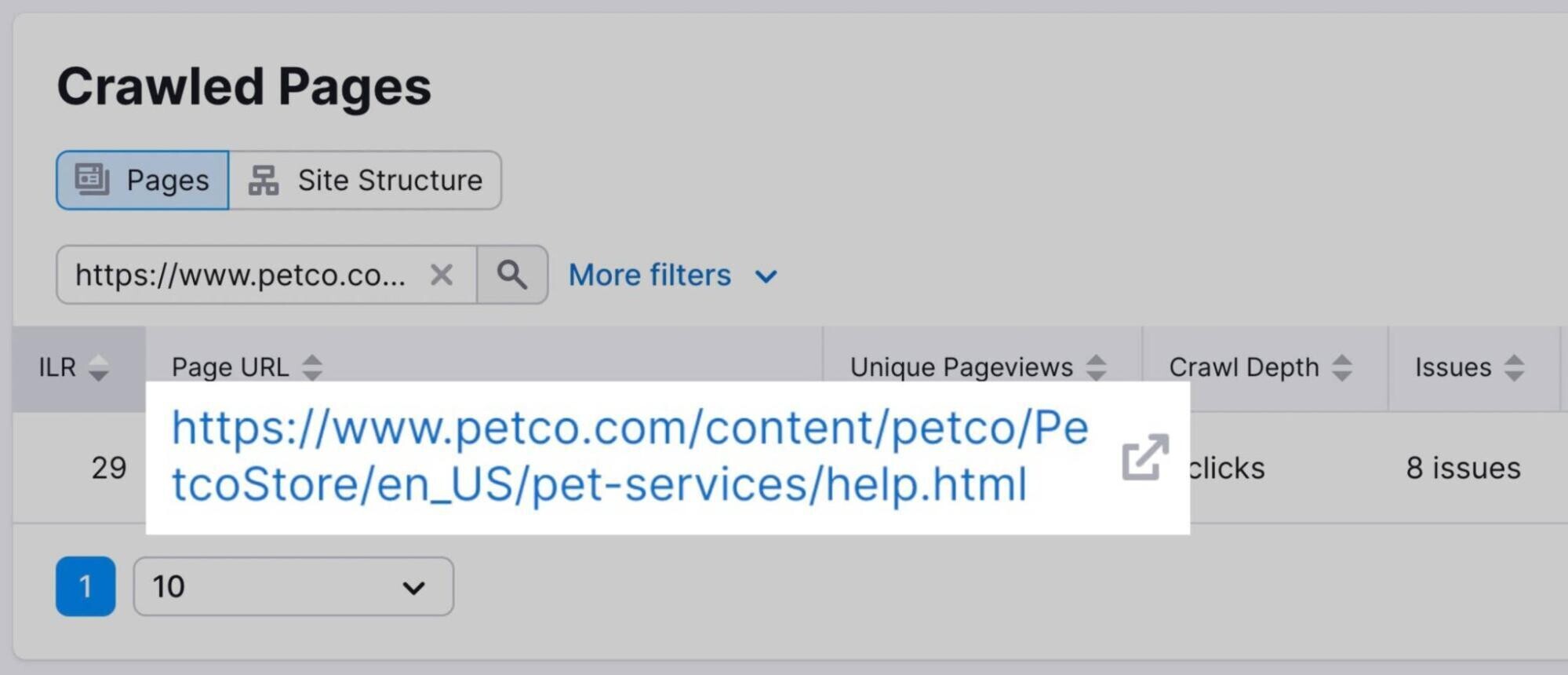
Below the “Incoming Inside Hyperlinks” part, you’ll discover a checklist of inside hyperlinks that direct to your outdated URL.
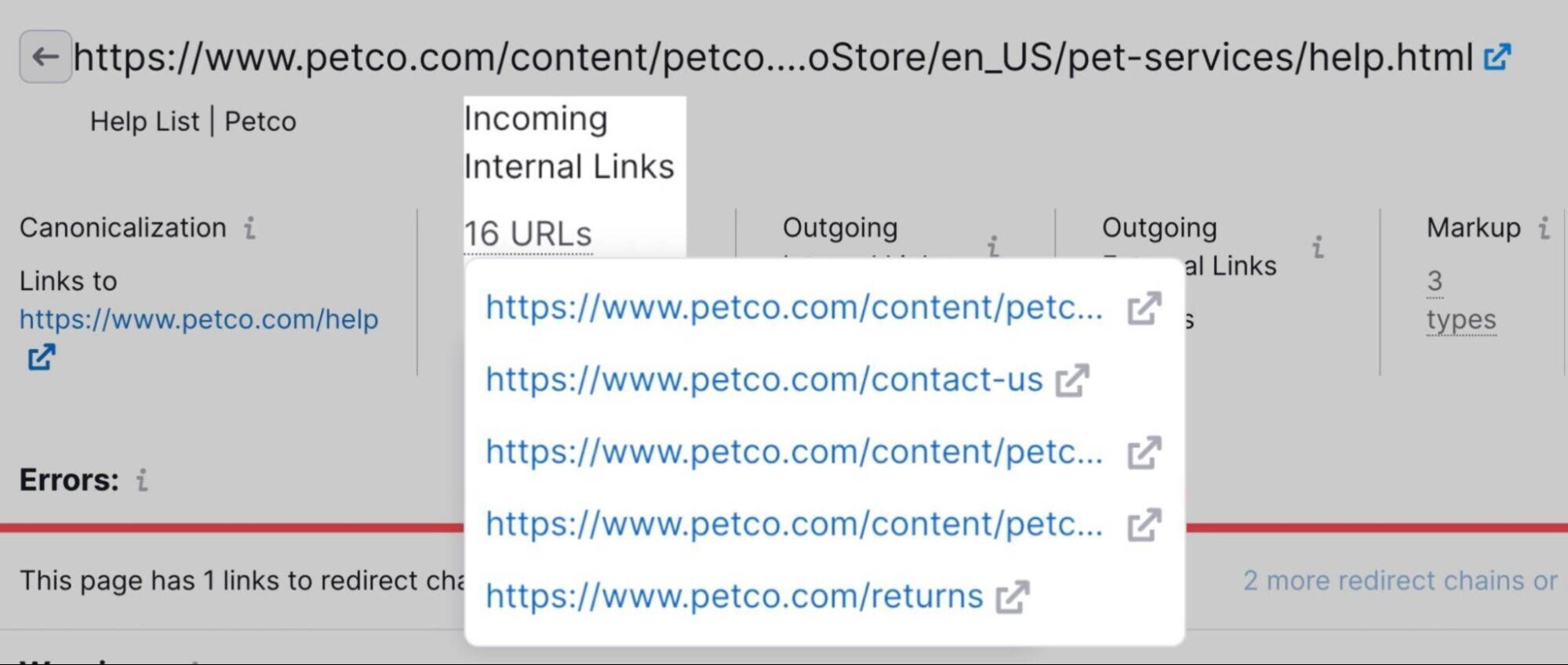
To keep away from pointless redirects, all it’s good to do is change the outdated hyperlink with the brand new hyperlink.
How?
You’ll have to do that by going to every web page and manually altering the hyperlink.
JavaScript Redirect FAQs
What Is a JavaScript Redirect?
A JavaScript redirect is a technique that makes use of JavaScript to direct a browser from the present webpage to a distinct URL. It is sometimes used for conditional navigation or consumer interaction-triggered modifications, although it requires the consumer’s browser to allow JavaScript.
Are Redirects with JavaScript Good or Unhealthy For website positioning?
JavaScript redirects can be utilized with out essentially harming website positioning however are typically much less favored than server-side redirects. This is because of their dependence on JavaScript being enabled on the consumer’s browser and their potential to be neglected by engines like google in the course of the crawling and indexing course of.
What’s the Greatest Different to a JavaScript Redirect?
The perfect different is to make use of server-side redirect strategies, equivalent to 301 redirects, that do not depend on JavaScript and supply express directions that engines like google higher perceive.
Wish to study extra about JavaScript and redirects?
Take a look at these sources:
[ad_2]
Source link